Simple YouTube Clone Created With Python And Django
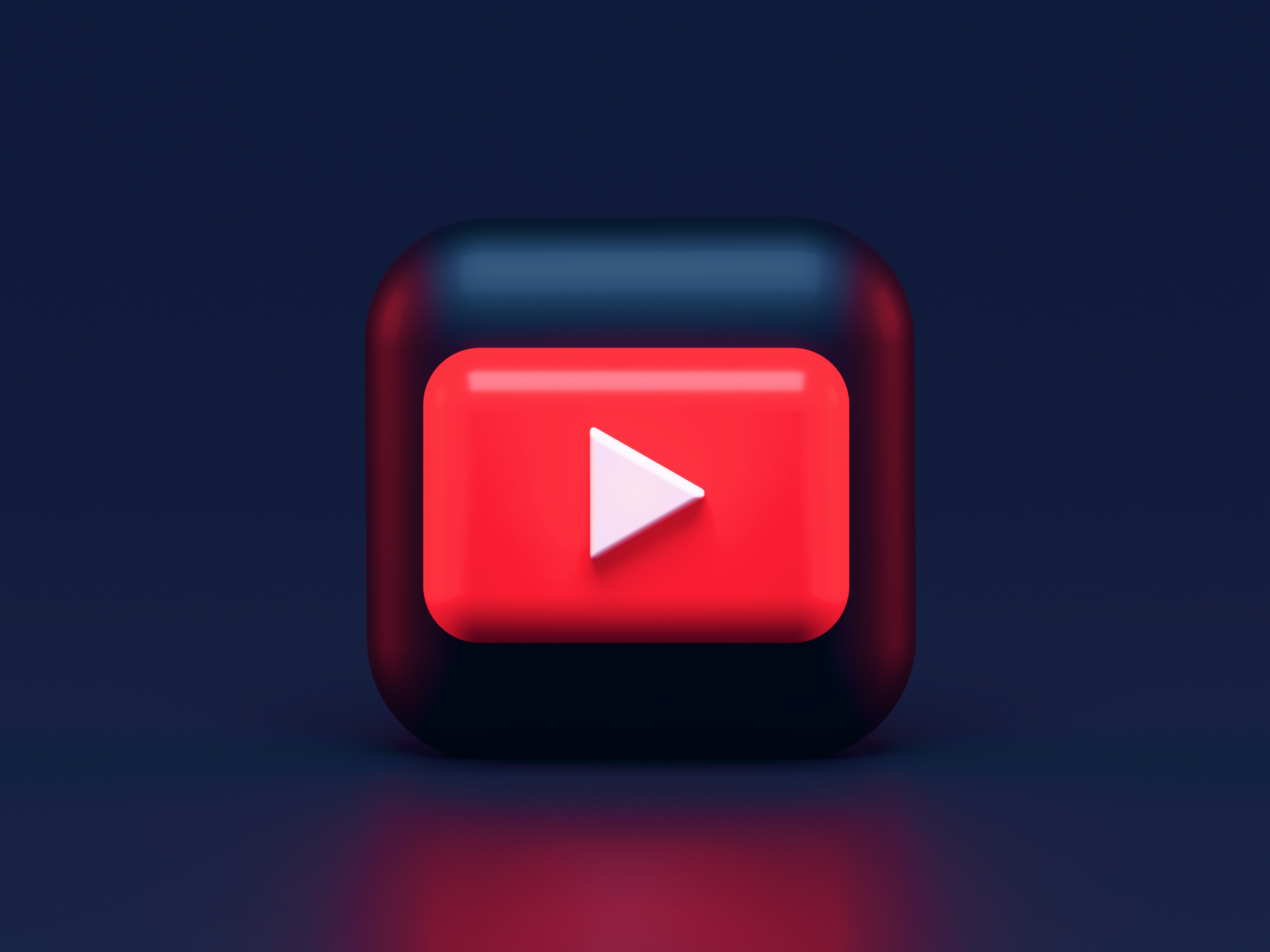
So far I have been using simple Django projects to explain things on this blog, now it is time to try something different. I have been thinking of a project that is exciting , a project that will make a beginner want to learn Django.
After doing my research, I settled on a project that everybody is familiar with, something people use on a daily basis and that project was a YouTube clone. I created a simple YouTube clone , it does not have all the features of YouTube but it has the following features.
Below are the Screenshots
You can watch videos just like YouTube
There is simple recommended videos on the side
You can comment on videos
Users have their own profile
Users can edit video
Users can login/logout
You can find this project on github here Vidakali
If you are a beginner, I advice you to take this project and try to modify a few things, maybe you could start by changing its name or adding new features to it . Feel free to do anything to it.
What You Will Learn
In this project you will learn how custom user model works in Django.
Custom User Model in Django
class CustomUserManager(BaseUserManager):
def create_user(self, username, email, password=None):
if not email:
raise ValueError('The Email must be set')
user = self.model(username=username,
email=self.normalize_email(email)
)
user.set_password(password)
user.save(using=self._db)
return user
def create_superuser(self, username, email, password=None):
user = self.create_user(
username, email, password=password
)
user.is_admin = True
user.is_staff = True
user.save(using=self._db)
return user
class CustomUser(AbstractBaseUser):
username = models.CharField(max_length=300, unique=True)
email = models.EmailField(
max_length=255,
unique=True,
verbose_name='email address')
avatar = models.ImageField(upload_to='avatars/', null=True, blank=True)
is_admin = models.BooleanField(default=False)
is_staff = models.BooleanField(default=False)
objects = CustomUserManager()
USERNAME_FIELD = 'email'
REQUIRED_FIELDS = ['username']
def __str__(self):
return self.email
def get_short_name(self):
# The user is identified by their email address
return self.email
def has_perm(self, perm, obj=None):
"Does the user have a specific permission?"
# Simplest possible answer: Yes, always
return True
def has_module_perms(self, app_label):
"Does the user have permissions to view the app `app_label`?"
# Simplest possible answer: Yes, always
return True
Also note we have USERNAME_FIELD = 'email'
, our unique identifier will be email
,
you will also learn how django does form field validation.
Cleaning a Specific Field in Django
class VideoForm(forms.ModelForm):
. . .
class Meta:
model = Video
fields = ['post', 'video_file']
def clean_video_file(self):
VIDEO_FILE_TYPES = ['mp4']
uploaded_video = self.cleaned_data.get("video_file", False)
extension = str(uploaded_video).split('.')[-1]
file_type = extension.lower()
if not uploaded_video:
raise forms.ValidationError("please upload a video")
if file_type not in VIDEO_FILE_TYPES:
raise forms.ValidationError("Upload an mp4 file")
return uploaded_video
Here we want users to upload mp4 files, anything else will be rejected and it will throw an error.
How to Upload Files Using Django and JavaScript
In this project you will learn how you can use Django and JavaScript to upload files, I have tried to use Vanilla JS
as much as possible. The only place I have used JQuery
is in opening bootstrap modal and closing. The alternative Vanilla JS
version of opening modal is complex with bootstrap 4.
document.addEventListener("click", function(e) {
if(e.target.id == "video_submit" ) {
let formdata = new FormData();
let video_file = document.getElementById('id_video_file').files[0];
formdata.append('video_file', video_file );
let csrf = document.querySelector('input[name="csrfmiddlewaretoken"]').value;
formdata.append('csrfmiddlewaretoken', csrf);
let post = document.querySelector('input[name="post"]').value;
formdata.append('post', post)
fetch('/videos/add_video/', {
method: 'POST',
mode: 'same-origin',
headers:{
'Accept': 'application/json',
'X-Requested-With': 'XMLHttpRequest',
'X-CSRFToken': csrf,
},
body: formdata
})
.then(response => {
return response.json()
})
.then(data => {
if (!data.form_is_valid){
document.getElementById("modal-content").innerHTML = " ";
document.getElementById("modal-content").innerHTML = data.video_form;
} else if (data.form_is_valid) {
$("#staticBackdrop").modal("hide");
location.reload();
}
})
}
});
You will see how we used JavaScript Fetch API to upload files with Django, previously people used to use jQuery.ajax().
I just want to leave this quote here
" If I have seen further than others, it is by standing upon the shoulders of giants. "
One of those is Mr Vitor Freitas, other resources I have used include
Header photo by Alexander Shatov
Keep Learning
- Django Translation To Different Languages Example
- Django Custom Template Filter
- Chains, Groups and Chords in Celery