What Is The Difference between AbstractUser and AbstractBaseUser in Django ?
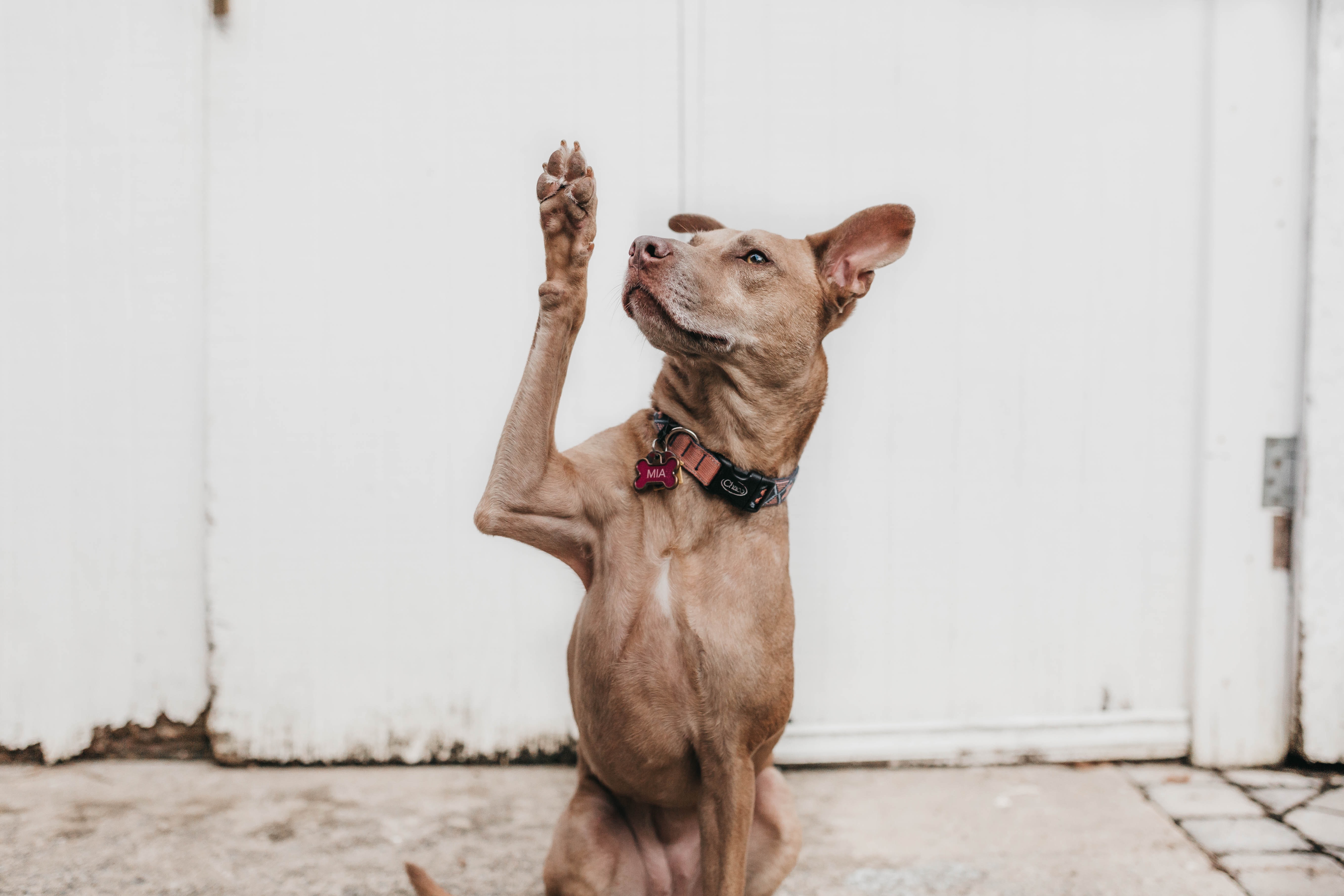
It is important to understand the difference between AbstractUser and AbstractBaseUser in Django, this will help you make the right decision on which one to use when you are starting a Django project.
AbstractUser
Django documentation says that AbstractUser provides the full implementation of the default User as an abstract model, which means you will get the complete fields which come with User model plus the fields that you define.
Example
from django.db import models
from django.contrib.auth.models import AbstractUser
class MyUser(AbstractUser):
address = models.CharField(max_length=30, blank=True)
birth_date = models.DateField()
In the above example, you will get all the fields of the User model plus the fields we defined here which are address and birth_date
AbstractBaseUser
AbstractBaseUser has the authentication functionality only , it has no actual fields, you will supply the fields to use when you subclass.
You also have to tell it what field will represents the username, the fields that are required, and how those users will be managed.
Lets say you want to use email in your authentication , Django normally uses username in authentication, so how do you change it to use email ?
Example
from django.db import models
from django.contrib.auth.models import AbstractBaseUser
class MyUser(AbstractBaseUser):
email = models.EmailField(
verbose_name='email address',
max_length=255,
unique=True,
)
date_of_birth = models.DateField()
is_active = models.BooleanField(default=True)
is_admin = models.BooleanField(default=False)
objects = MyUserManager()
USERNAME_FIELD = 'email'
REQUIRED_FIELDS = ['date_of_birth']
USERNAME_FIELD is a string describing the name of the field on the user model that is used as the unique identifier.
In the above example, the field email is used as the identifying field.
It is important to note that you can also set email as your unique identifier by using AbstractUser, this can be done by setting username = None
and USERNAME_FIELD = 'email'
as shown below
Example
from django.db import models
from django.contrib.auth.models import AbstractUser
class MyUser(AbstractUser):
username = None
email = models.EmailField(
verbose_name='email address',
max_length=255,
unique=True,
)
USERNAME_FIELD = 'email'
REQUIRED_FIELDS = []
objects = MyUserManager()
def __str__(self):
return self.email
Now which one should you use ?
If you need full control over User model, it is better to use AbstractBaseUser but if you are just adding things to the existing user, for example, you just want to add an extra field e.g location field or any other profile data then use AbstractUser
RESOURCES
Header photo by Camylla Battani on unsplash
Customizing authentication in Django
Difference of customization user model between AbstractBaseUser and AbstractUser
Keep Learning
- Django Translation To Different Languages Example
- Django Authentication Tutorial
- How to send SMS using Django