django messages framework example
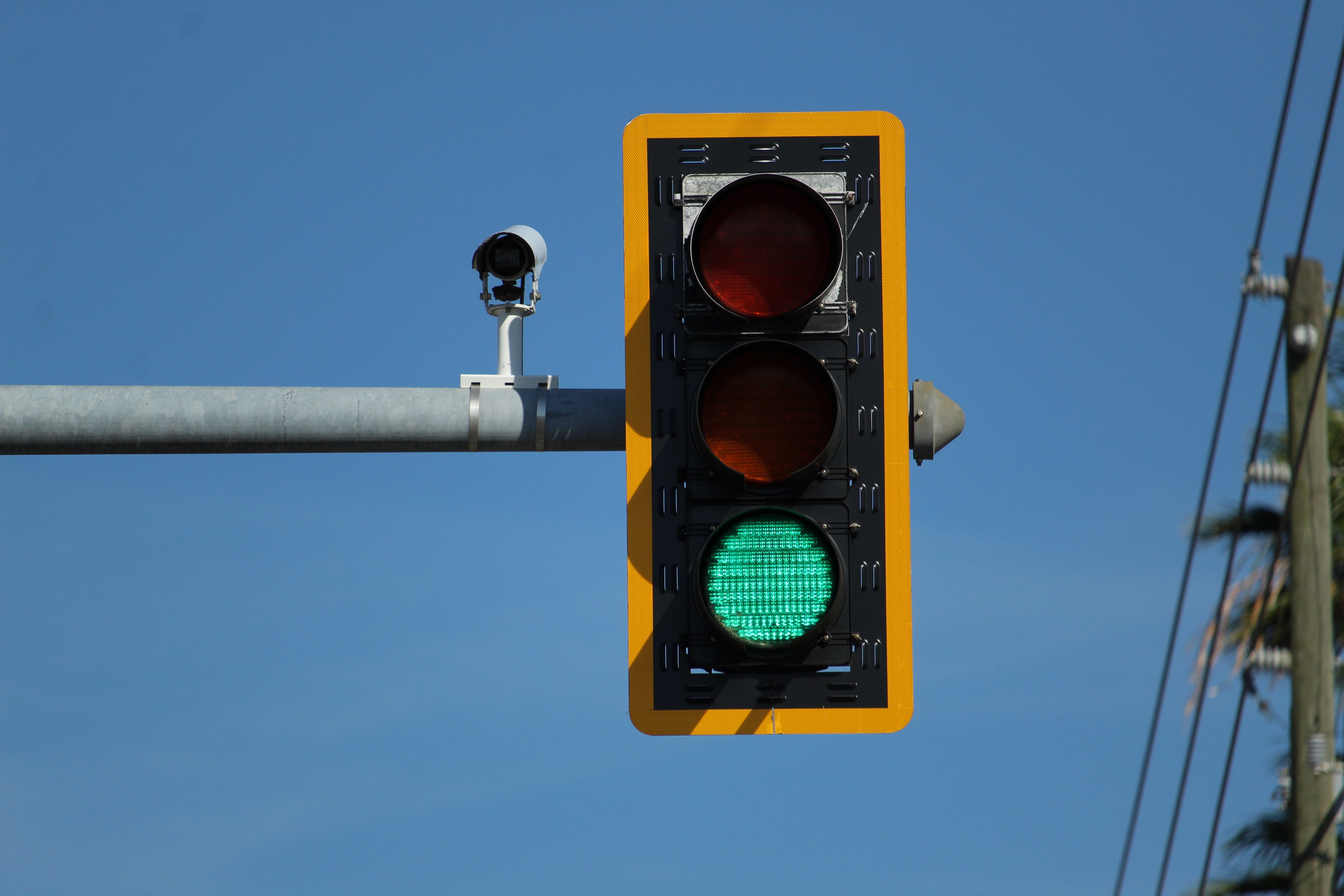
Django messages framework provides a way of notifying users that a certain action was done. For example when you are changing your password, you might get this message "your password was changed successfully" . The message is important because it assures the user that indeed a certain action was done successfully. Django documentation says that
The messages framework allows you to temporarily store messages in one request and retrieve them for display in a subsequent request (usually the next one). Every message is tagged with a specific level that determines its priority (e.g., info, warning, or error).
How do we enable messages ?
Django messages framework is enabled by default, so you do not need to change anything in settings.py
file.
Message levels
The message levels help us in grouping messages by type, we have the following message levels.
Constant Purpose
DEBUG
Development-related messages that will be ignored
(or removed) in a production deployment.
INFO
Informational messages for the user .
SUCCESS
An action was successful, e.g. “Your profile was updated successfully” .
WARNING
A failure did not occur but may be imminent.
ERROR
An action was not successful or some other failure occurred.
Message tags
Message tags are string representation of the message levels, they are used as CSS classes and they will help in styling different messages. For example you might want warning messages to appear as red in color and success message to appear as green, message tags will help you in achieving that.
Level Constant Tag
DEBUG
debug
INFO
info
SUCCESS
success
WARNING
warning
ERROR
error
Using messages in views
In this example we will add a success message when the image is uploaded successfully. checkout the full source code of this example app here
def model_form_upload(request):
if request.method == 'POST':
form = DocumentForm(request.POST, request.FILES)
if form.is_valid():
form.save()
messages.success(request, 'your image was uploaded successfully') # added success message here
return redirect('home')
else:
form = DocumentForm()
return render(request, 'uploads/model_form_upload.html', {
'form': form
})
and then in the template you can display the message like this
{% if messages %}
<ul class="messages">
{% for message in messages %}
<li{% if message.tags %} class="{{ message.tags }}"{% endif %} > {{ message }} </li>
{% endfor %}
</ul>
{% endif %}
This was a very simple example of how Django's messages framework works. For more information on this topic checkout Vitor Freitas tutorial on the same topic, he covers a lot , from adding bootstrap to custom message tags.
Resources
Keep Learning
- How to solve “TemplateDoesNotExist” error in Django
- What is super() in python
- How Django Logging Works