What Is Select_related in Django
July 19, 2021, 9:19 a.m.
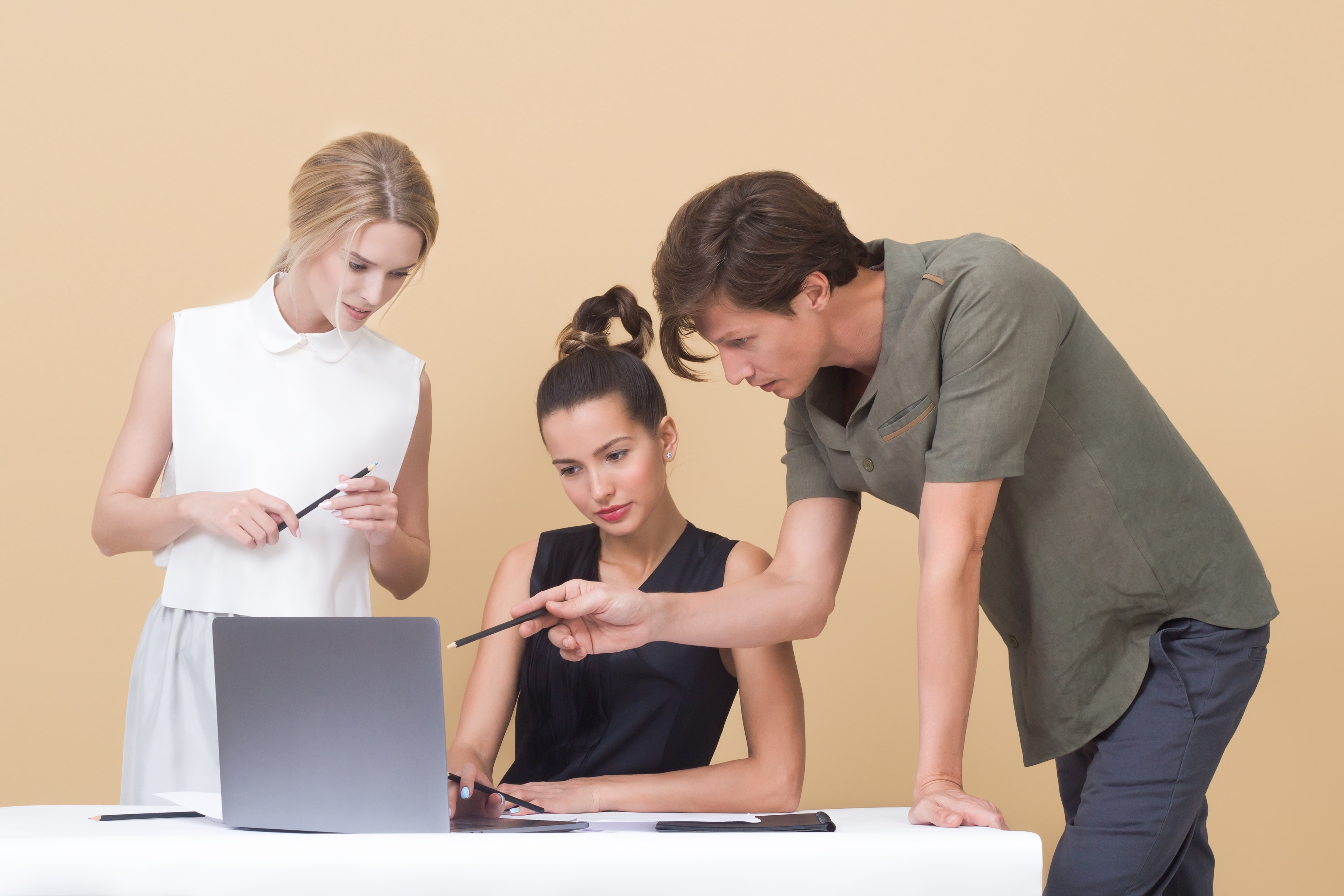
select_related() returns a QuerySet that follows foreign-key relationships, selecting additional related-object data when it executes its query. It creates a single complex query which means later use of foreign-key relationships will not hit the database which leads to a performance boost.
Example:
We will use the following models to demonstrate how it works
class Person(models.Model):
first_name = models.CharField(max_length=100)
last_name = models.CharField(max_length=100)
birthdate = models.DateField()
def __str__(self):
return self.first_name
class Book(models.Model):
name = models.CharField(max_length=100)
author = models.ForeignKey(Person, on_delete=models.CASCADE)
def __str__(self):
return self.name
if you want to get the author from Book instance, there are two ways of doing this, the first way is shown below
book = Book.objects.get(pk=5) # Hits the database
author = book.author # Hits the database again
And here’s select_related lookup:
# Hits the database.
book = Book.objects.select_related('author').get(pk=5)
# Doesn't hit the database, because book.author has been prepopulated
# in the previous query.
author = book.author
Using the second example is more efficient because we don't have to query the database twice, this is why we say select_related is a performance booster.
Resources
Header photo by Icons8 Team on Unsplash
Keep Learning
- Difference between Django forms.Form and forms.ModelForm
- Custom Managers and QuerySet Methods in Django
- Chains, Groups and Chords in Celery