Difference between Django forms.Form and forms.ModelForm
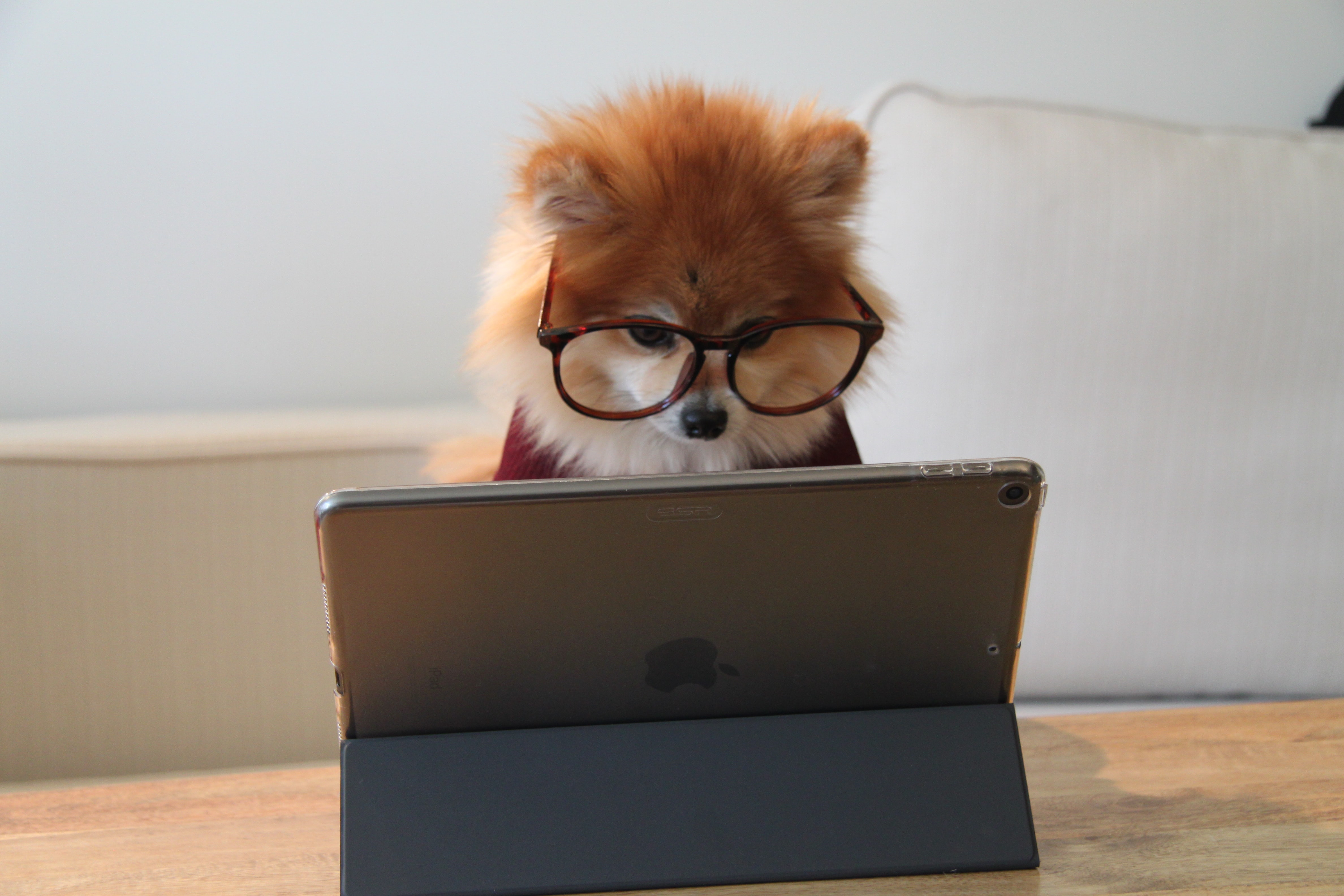
This article assumes you have worked with forms in your Django projects before. In this article we are going to look at the difference between Django forms.Form
and forms.ModelForm
.
A Form created from forms.ModelForm
is a Form that is automatically created based on a particular model
example
lets say you have an article model that looks like this
class Article(models.Model):
DRAFT = 'D'
PUBLISHED = 'P'
STATUS = (
(DRAFT, 'Draft'),
(PUBLISHED, 'Published'),
)
title = models.CharField(max_length=255)
content = models.TextField(max_length=4000)
status = models.CharField(max_length=1, choices=STATUS, default=DRAFT)
create_date = models.DateTimeField(auto_now_add=True)
update_date = models.DateTimeField(blank=True, null=True)
In your forms.py file you can create your forms automatically like so
from django import forms
from . import models
class ArticleForm(forms.ModelForm):
class Meta:
model = models.Article
fields = ['title', 'content', 'status']
A model form must have a model to work from, in the above example you can see this line " model = models.Article
" here we are specifying the model that this form will be based on
Django documentation says that
In fact if your form is going to be used to directly add or edit a Django model, a ModelForm can save you a great deal of time, effort, and code, because it will build a form, along with the appropriate fields and their attributes, from a Model class.
Now lets look at forms created from forms.Form
Forms created from forms.Form
are forms which are independent of a model and do not directly interact with models.
An example can be a search form
from django import forms
class SearchForm(forms.Form):
search_term = forms.CharField(required=False, max_length=50)
As you can see, the above SearchForm is not tied to a particular model , it is independent and its sole purpose is to generate fields or a field if you have only one field like our search form above which will be presented to the end user .
The main difference between the two is that in forms that are created from forms.ModelForm
, we have to declare which model will be used to create our form.
In our Article Form above we have this line " model = models.Article
" which basically means we are going to use Article model to create our form.
Whereas in Forms created from forms.Form
like our SearchForm above, that line is missing , and that is the reason why we say forms created from forms.Form
are independent and do not depend on any model to create the form fields.
Resources
Keep Learning
- What Is The Difference between AbstractUser and AbstractBaseUser in Django ?
- What is __init__() in Python
- Python Django Web Scraping