Django Custom Template Filter
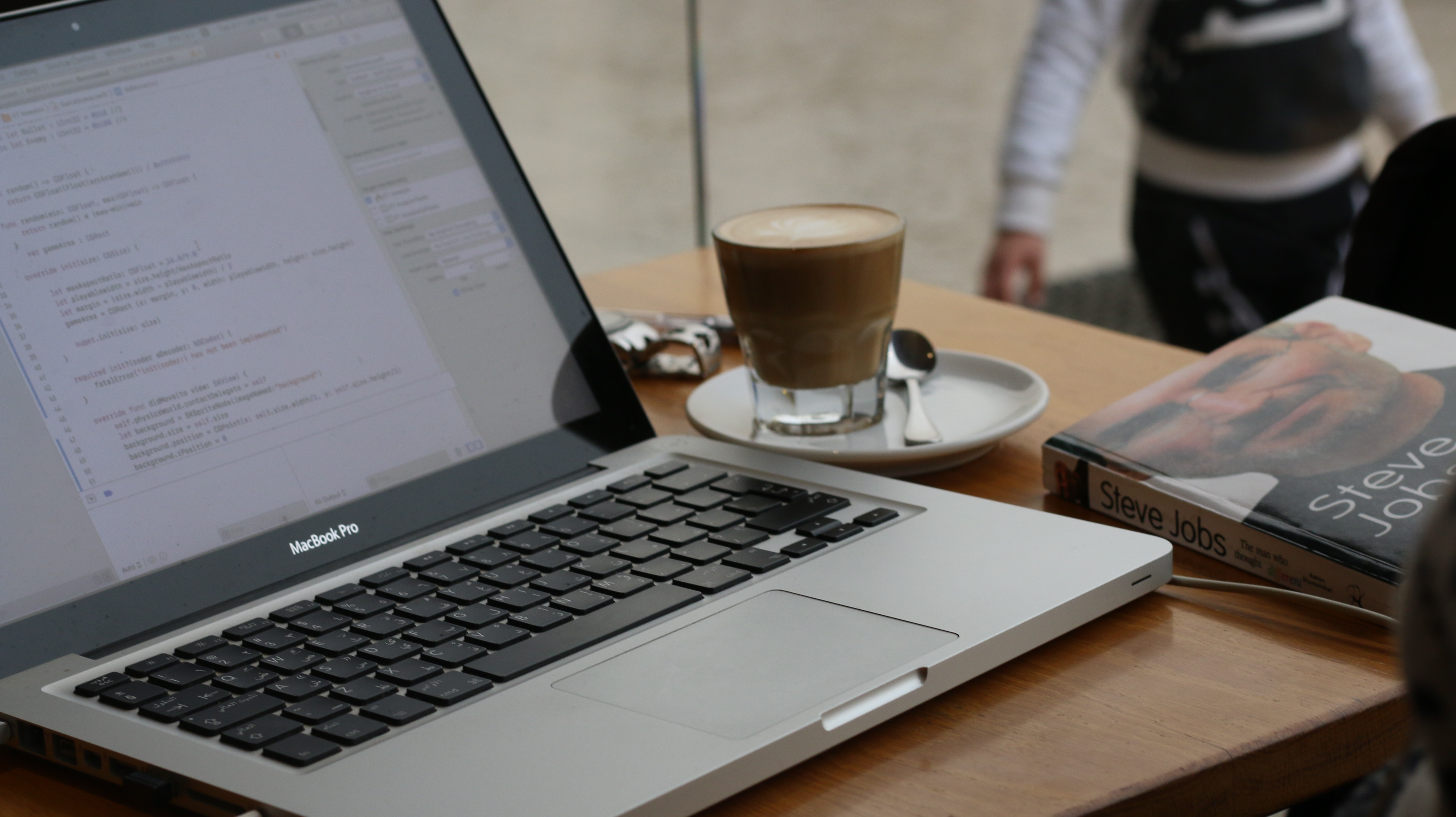
We are going to create a custom template filter in this tutorial, we will create a simple template filter that converts regular numbers to a cool format for example 2000 will be 2k and 1000000 becomes 1M , this format is used by companies like twitter and instagram to calculate the number of followers their users have.
We are going to be using the python function below to do the conversion for us
def human_format(num):
decimal_format = '{:.2f}'
int_num = int(num)
if int_num > 999 and int_num < 1000000:
return decimal_format.format(int_num/1000.0).rstrip('0').rstrip('.') + 'K'
elif int_num > 999999:
return decimal_format.format(int_num/1000000.0).rstrip('0').rstrip('.') + 'M'
elif int_num < 1000:
return str(int_num)
Writing custom template filters
-
Create a file named templatetags inside your app on the same level as models.py and views.py.
-
Inside templatetags folder create an empty
__init__.py
file to ensure the directory is treated as a Python package. -
Create a module inside templatetags folder and name it what you want , lets say we decide to name it
custom_filter.py
. The name of the module file will be the name you’ll use to load the tags later, so in our example we will load our custom filter in our template like so {% load custom_filter %}
The code inside our custom_filter.py
will look as shown below
from django import template
register = template.Library()
def human_format(num):
decimal_format = '{:.2f}'
int_num = int(num)
if int_num > 999 and int_num < 1000000:
return decimal_format.format(int_num/1000.0).rstrip('0').rstrip('.') + 'K'
elif int_num > 999999:
return decimal_format.format(int_num/1000000.0).rstrip('0').rstrip('.') + 'M'
elif int_num < 1000:
return str(int_num)
register.filter('human_format', human_format )
This line register.filter('human_format', human_format ) is used to register our custom template filter.
register.filter( ) takes in two arguments:
- The name of the filter
- The compilation function
USING OUR CUSTOM FILTER
You can use it in your template like this
{% load custom_filter %}
<p> Joe biden has over {{num_followers|human_format}} followers </p>
Assume the value of num_followers is 29700000, Using our custom filter, this will translate to Joe biden has over 29.7M followers .
SOURCE CODE
https://github.com/felix13/django_custom_template_filter.git
RESOURCES
Custom template tags and filters
Header photo by Abdelrahman Sobhy
Keep Learning
- Simple Pagination in Django
- Difference between Django forms.Form and forms.ModelForm
- Custom Managers and QuerySet Methods in Django