Django Class Based Views vs Function Based Views
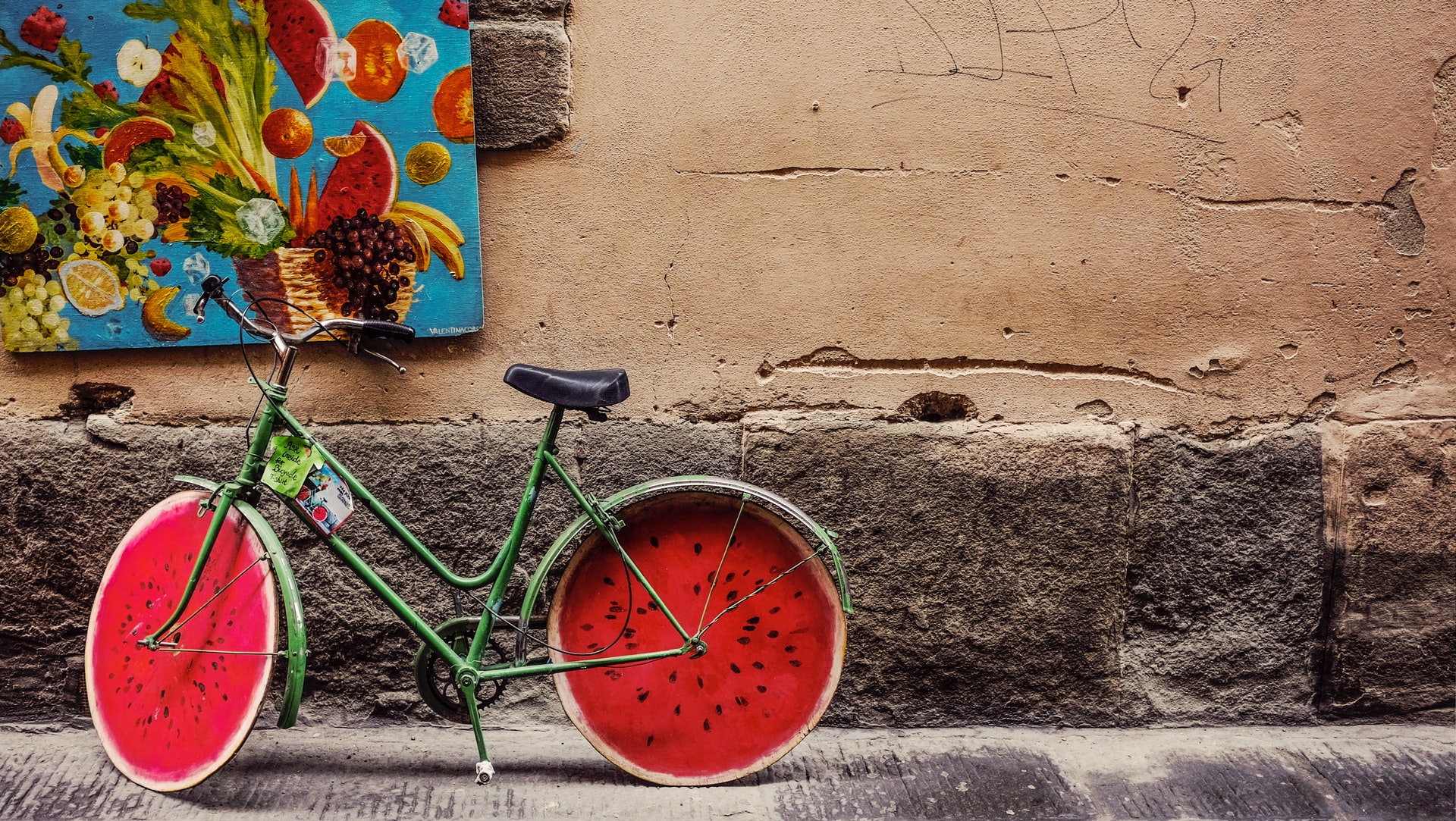
In this blog post, we will cover what is the difference between class-based views and function-based views in Django. we are going to see the pros and cons of each approach.
Introduction
In the beginning, Django only had function-based views but they were hard to extend or customize beyond some configuration options. This led to the introduction of class-based views which were now easier to extend using mixins compared to function-based views.
It is important to understand that class-based views are not a replacement for function-based views. If you are a beginner, make sure you understand how python classes and functions work first before jumping into Django. A very simple Django view might look like
from django.http import HttpResponse
def MyView(request):
if request.method == 'GET':
return HttpResponse('response')
If you are new to Django, this code normally lives in the views.py file in a Django project. In Django, views normally take in a request and return a response. If you look at the above code, you will see we are checking if the method is a GET request, if that is true, we return a response. All these were done using a function and that is why it is called a function-based view. Now let's look at the equivalent of doing the same using a class, the code for that might look like
from django.http import HttpResponse
from django.views import View
class MyView(View):
def get(self, request):
return HttpResponse('response')
As you can see in the above code, we are using a class to achieve the same thing we achieved using the function-based view. Here we are returning a response if the method is a GET request. When we use a class in a view, we can say we are using class-based views.
When using class-based views, handling of HTTP methods (GET, POST, etc.) is done using separate methods instead of conditional branching.
Adding context to a template
If you want to add context to the template using a function-based view, let's say you want to pass age to the template. You can do that with the function below
def home(request):
context = {
'age': 25,
}
return render(request, "home.html", context )
you can see with a function-based view it's simple, now let's try doing the same using a class-based view
from django.views.generic import TemplateView
class HomePageView(TemplateView):
template_name = "home.html"
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
context['age'] = 25
return context
The above example is not clear if you are new to Django, this is because you need to know the methods available and the specific method to override to achieve what you want. In the above code, we have used a class-based view to add context to the template. Our context is an age that is set to 25.
The URL pattern for this might look like
urlpatterns = [
path('', HomePageView.as_view(), name='home'),
]
Updating Objects with Function-Based Views
Let's look at how to update objects using a function-based view, this can be done as shown below
def edit(request, id):
article = get_object_or_404(Article, pk=id)
if request.method == 'POST':
form = ArticleForm(request.POST, instance=article)
if form.is_valid():
form.save()
return redirect('/articles/')
else:
form = ArticleForm(instance=article)
return render(request, 'articles/edit.html', {'form': form})
With a function-based view, you can see and follow exactly what is going on. When you use class-based views that is not the case.
Updating Objects with Class-Based Views
Updating objects with class-based views might be as simple as shown below.
class ArticleUpdate(LoginRequiredMixin, UpdateView):
template_name = 'articles/edit.html'
model = Article
form_class = ArticleForm
success_url = reverse_lazy('articles')
You can see with class-based views we are using less code but now you cannot see what is going on and it feels like magic for people who are new to Django.
Class-based views
pros:
- When you are using class-based views you can re-use code
- It is easy to follow the DRY (Don't repeat yourself) principle with class-based views by using object-oriented techniques such as multiple inheritance.
- Handling of specific HTTP methods (GET, POST, etc.) is done using separate methods instead of conditional branching.
cons:
- It is not easy to see exactly what is going on especially if you are a beginner.
Function based views
pros:
- They are easy to read and understand, everything is clear and straightforward.
- It is easier to use decorators with function-based views.
cons:
- It is not easy to re-use code
Personal Preference
I prefer function-based views because of their simplicity, with function-based views you can see exactly what is going on. This does not mean that class-based views are a bad option, in fact, class-based views sometimes come in handy when you need to re-use code in your project.
Conclusion
We have seen the pros and cons of function-based views and class-based views, in my opinion, I think it's important to understand how both class-based views and function-based views work. If you are a beginner, you could start with function-based views because they are easier and after you get comfortable you can move to class-based views.
Resources
- Header photo by Cristina Gottardi on Unsplash
- Django documentation on Class-based views
- Django Views — The Right Way
Keep Learning
- How to Serve Django's Static Files with NGINX on Localhost
- How Does Django and Celery Work
- How Django Signals Work